normalise#
- moocore.normalise(data, /, to_range=[0.0, 1.0], *, lower=nan, upper=nan, maximise=False)[source]#
Normalise points per coordinate to a range, e.g.,
to_range = [1,2]
, where the minimum value will correspond to 1 and the maximum to 2.- Parameters:
data (
ArrayLike
) – Numpy array of numerical values, where each row gives the coordinates of a point in objective space.to_range (
ArrayLike
, default:[0.0, 1.0]
) – Range composed of two numerical values. Normalise values to this range. If the objective is maximised, it is normalised to(to_range[1], to_range[0])
instead.upper (
ArrayLike
, default:nan
) – Bounds on the values. Ifnumpy.nan
, the maximum and minimum values of each coordinate are used.lower (
ArrayLike
, default:nan
) – Bounds on the values. Ifnumpy.nan
, the maximum and minimum values of each coordinate are used.maximise (
bool
|list
[bool
], default:False
) – Whether the objectives must be maximised instead of minimised. Either a single boolean value that applies to all objectives or a list of booleans, with one value per objective. Also accepts a 1D numpy array with values 0 or 1 for each objective
- Returns:
ndarray
– Returns the data normalised as requested.
Examples
>>> dat = np.array([[3.5, 5.5], [3.6, 4.1], [4.1, 3.2], [5.5, 1.5]]) >>> moocore.normalise(dat) array([[0. , 1. ], [0.05 , 0.65 ], [0.3 , 0.425], [1. , 0. ]])
>>> moocore.normalise(dat, to_range=[1, 2], lower=[3.5, 3.5], upper=5.5) array([[1. , 2. ], [1.05, 1.3 ], [1.3 , 0.85], [2. , 0. ]])
Gallery examples#
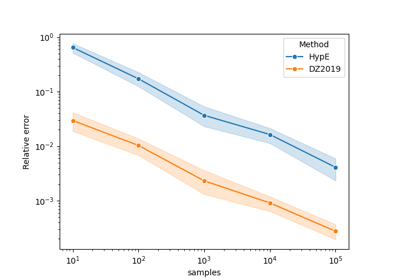
Comparing methods for approximating the hypervolume